Flutter onGenerateRoute is alternative for routes property of MaterialApp and it’s an alternative for using any plugins for route. Not only that, it also works as a middleware for routes.
In general when we use routes property we assign a map or routes to it. It works with pushNamed or named route.
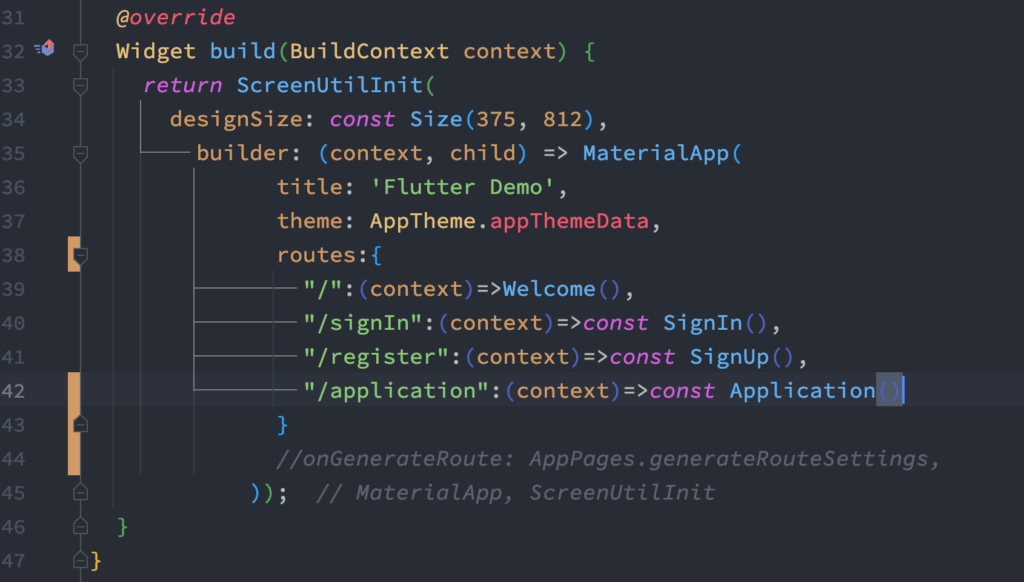
See that we assign routes property with a Map. But this could become huge items of Map and not sustainable.
The alternative and better approach is using onGenerateRoute property of MaterialApp. It takes a callback function with MaterialPageRoute return type.
Again it means onGenerateRoute will take a function and the function should return MaterialPageRoute.
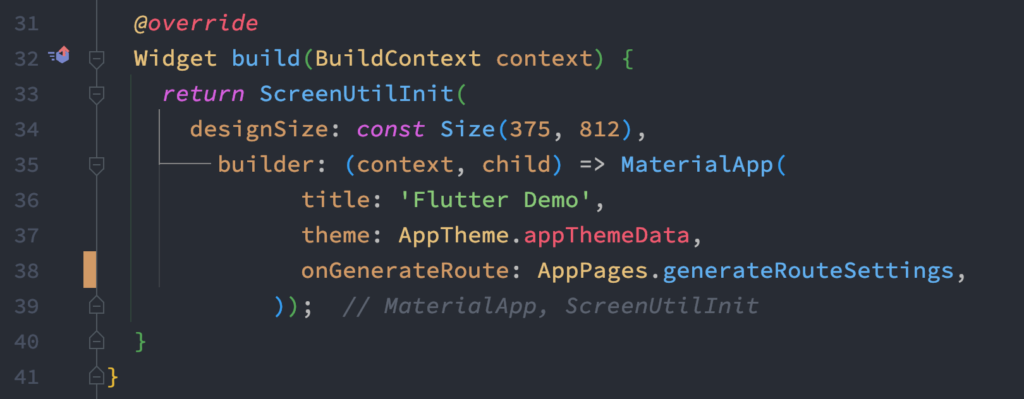
See from line 38 that, we have onGenerateRoute and there’s an assignment of a callback function. Now let’s take look at our the callback.
static MaterialPageRoute generateRouteSettings(RouteSettings settings) {
if (kDebugMode) {
print("clicked route is ${settings.name}");
}
if(settings.name!=null){
var result = routes().where((element) => element.path==settings.name);
if(result.isNotEmpty){
//if we used this is first time or not
bool deviceFirstTime= Global.storageService.getDeviceFirstOpen();
if(result.first.path==AppRoutesNames.WELCOME&&deviceFirstTime){
return MaterialPageRoute(
builder: (_) => const SignIn(),
settings: settings);
}else{
if (kDebugMode) {
print('App ran first time');
}
return MaterialPageRoute(
builder: (_) => result.first.page,
settings: settings);
}
}
}
return MaterialPageRoute(
builder: (_) => Welcome(),
settings: settings);
}
}
Here you must return MaterialPageRoute as your return type. And also see the RouteSettings class settings object.
The settings object can catch whatever the route gets triggered when you route by clicking pushNamed or named route.
You may see that from the last two return statements that we are returning MaterialPageRoute which returns a widget.
This widget could be a dart class for presenting a screen or page using stateful or stateless class.
I put many conditional checking in the callback. So here, It also works like a middleware in flutter. Here I have checked if we have the device or the app opened for the first time or not. If the app was opened before, then we go to sign_in routes or screen.